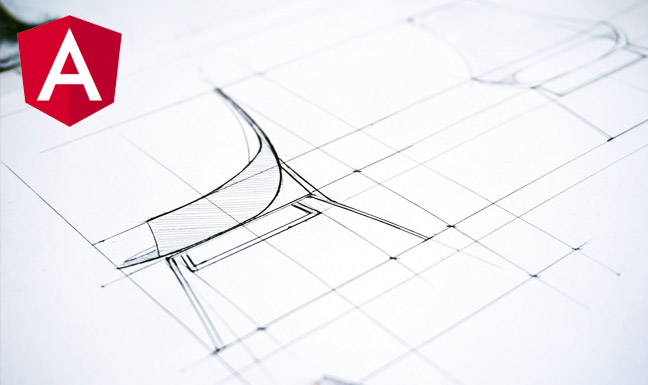
TypeScript Models
Basic overview of the TypeScript and ECMAScript 6 models. An example of use case to exemplify the use of models in external classes.
In the Angular world, the concept of data binding can be translated into communication. It defines the communication between the logic part of a component on a typescript file and the html template which will be rendered on the browser. There are four main types of data binding in an Angular app:
Consists of a basic rendering of data stored in a variable or the returning value of a function.
export class AppComponent {
headline: string;
constructor() {
this.headline = 'Title of the Headline';
}
}
// On the template, the output 'title of the Headline' is expected to be rendered
<h1>{{headline}}</h1>
Used for binding property data into a DOM element. The result of the binding will update each time Angular triggers its change detector. In the following snippet, both lines will have the same result:
<img src="{{ logoUrl }}">
<img [src]="logoUrl">
Allows a component to react to certain events or interactions from the template by executing a certain method defined on the script. The following example triggers the submitForm() method after the user clicks the button.
<button (click)="submitForm()">
List of supported events triggered by Angular on its change detection:
(focus)='method()'
(blur)='method()'
(submit)='method()'
(scroll)='method()'
(cut)='method()'
(copy)='method()'
(paste)='method()'
(keydown)='method()'
(keypress)='method()'
(keyup)='method()'
(mouseenter)='method()'
(mousedown)='method()'
(mouseup)='method()'
(click)='method()'
(dblclick)='method()'
(drag)='method()'
(dragover)='method()'
(drop)='method()'
Consists of a combination of Event Biding and Property Biding. It allows instant communication between the view and the model.
<input type="text" name="Name" [(ngModel)]="username">
<p>Your Name is: {{username}}!</p>
The previous example binds the value of an input to a component property named username. Then, it uses String Interpolation to render the value of that property inside a < p > element. When a user types on the input the value of username will change and the output text will change in 'real-time'.
Important!
In order to make use of the Two-Way Binding sintax, the ngModel directive must be enabled first. Do so by importing the module @angular/forms in the main app.module.ts file. Then add the FormsModule into the imports[] inside you AppModule.
import { FormsModule } from '@angular/forms';
@NgModule( {
imports: [
AppModule
]
} )
Basic overview of the TypeScript and ECMAScript 6 models. An example of use case to exemplify the use of models in external classes.
Access DOM elements directly using viewChild and Template Reference Variables.
Steps to compile an Angular application into a single bundle. An overview of the types of Angular compilation bundles and ways to cache bust an Angular application.