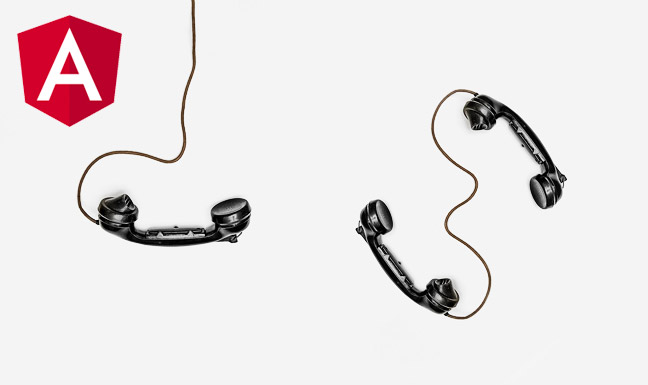
Angular: ViewChild and Local References
Access DOM elements directly using viewChild and Template Reference Variables.
Angular is one of the most advanced JavaScript frameworks at the moment. It is being maintained by a large community of engineers and powered by Google, giving that extra plus for reliability.
Its learning curve is often mentioned as a downside for this framework. However, once learnt its best practices and ways of developing it becomes one of the most solid tools for creating web applications.
Following the single-page application architecture, Angular apps are based on one fundamental piece: the router.
One of the keys to design a scalable Angular system is to modularize its sections. An Angular module consolidate components, directives, and pipes into cohesive blocks of functionality.
Every Angular app has at least one module, the root module. The rest of modules for organizing code are known as feature modules.
The routing of an application can be defined on a single routing module or be split into multiple routing modules. I find this really helpful as it allows an Angular system to scale very easily.
To see the nested routing in action, we can look at an example of Angular application that has 3 modules: MainModule
, UsersModule
and ConfigModule
.
This application will have the following three routes and each route will be defined as an independent module:/
/users
/config
It is simple to develop nested routers in Angular. The key is to rely on JavaScript’s dynamic imports of modules and Angular’s loadChildren
method.
This file is the main routing module. It defines the main routes while importing two nested modules.
const routes: Routes = [
{
path: '',
component: HomeComponent
},
{
path: 'users',
loadChildren: () => import('../modules/user/user.module')
.then(m => m.UsersModule),
},
{
path: 'config',
loadChildren: () => import('../modules/config/config.module')
.then(m => m.ConfigModule),
}
];
@NgModule({
imports: [RouterModule.forRoot(routes)],
exports: [RouterModule]
})
export class MainRoutingModule { }
The Angular application now has two nested modules: UsersModule
and ConfigModules
. These modules can then define their own routing with complete independence.
Example UsersModule
:
@NgModule({
declarations: [
UsersComponent,
UsersListComponent,
UsersSettingsComponent
],
imports: [
CommonModule,
UsersRoutingModule
]
})
export class UsersModule { }
Example UsersRoutingModule
:
const routes: Routes = [
{
path: '',
component: UsersComponent,
children: [
{
path: 'list',
component: UsersListComponent
},
{
path: 'settings',
component: UsersSettingsComponent
},
{
path: '',
redirectTo: 'list',
pathMatch: 'full'
}
]
}
];
@NgModule({
imports: [RouterModule.forChild(routes)],
exports: [RouterModule]
})
export class UsersRoutingModule { }
Access DOM elements directly using viewChild and Template Reference Variables.
Understand the basics of Angular routing and review core concepts and internal architecture.
Basic learning of how to use build-in tools provided by Angular in order to create components after an application has been loaded.