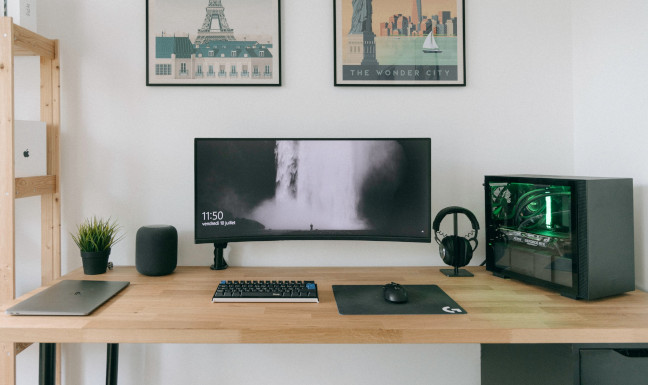
Top JavaScript Frameworks 2021
What are the top JavaScript Frameworks that you should be looking for in 2021? This article provides a list of the most commont frontend frameworks to use on a JavaScrtipt project.
React Hooks are functions that let you “hook into” React state and lifecycle features from function components. React Hooks are functions that facilitate the access and management of React’s state, props, context and lifecycles. They expose an API to React’s features that is easy to access.
React Hooks make React application easier to structure and improves code readability. Hooks can be used in React to reuse stateful logic without changing the component hierarchy. React exposes built-in Hooks but also allows you to build custom ones.
React Hooks helps to reduce code inconsistency. As opposed to the class based component’s lifecycle, hooks allow separation of logic. A component can be split into multiple functions based on the data they manage. The key to React Hooks is that they allow easy access to React’s features without using classes.
React Hooks are basic JavaScript functions. Hooks need to follow a couple of rules when using them in a project.
By following these rules, React ensures that manipulations to a component’s state will be visible to anywhere on the source code. To help developers, React provides a linter plugin to enforce these rules automatically in your code.
The React State Hook allows function components to set a local state that will be preserved between component renders. The value of the State Hook can be used after initialization for either plain JavaScript functions or as part of the HTML DOM inside the JSX template.
The useState Hook accepts a single parameter that defines the initial value of the local state. This value can be of any type: string, array, object and so. The return of the useState Hook is pair: a variable storing the current local state and a function that can be called to update the state.
As opposed to state in React classes, the React useState
Hook does not override the full state of a component. Instead, it only affects individual properties of the state. This means that a functional component can use multiple useState Hooks to handle state independently.
React State Hook works perfectly with the destructuring assignment syntax. This is a JavaScript expression that makes it possible to unpack values from arrays, or properties from objects, into distinct variables.
Here is an example of code code using multiple useState hooks.
const [active, setActive] = useState(true);
const [name, setName] = useState("user");
...
setActive(false);
The React Effect Hook, useEffect
, allows you to run logic after the component has rendered. They are perfect for handling external API calls, data fetching, subscriptions or DOM modifications after the initial load. React treads these executions as effects and exposes the Effect Hook to manage it.
As React Effect Hooks are defined inside a React functional component, they have access to the component’s state and props. When a component re-renders, React will run any Effect Hook defined within it.
React Effect Hooks allow you to define return functions that will be called when a component gets unmounted. This is very helpful to manage unsubscriptions and data clean up.
Similar to the React State Hooks, there can be multiple Effect Hooks inside a component. This allows, for example, to execute asynchronous logic in parallel while the application waits for external events to happen.
Here is an example of code using the React Effect Hook.
useEffect( () => {
// Perform action when active value changes.
}, [active]);
The React Reducer Hooks is an alternative to useState
. This React Hook is usually preferable to useState
when a React component has to deal with complex state logic involving multiple sub-values.
React Reducer Hook also lets you optimize a component’s performance as it returns a dispatch
instead of function callbacks. The benefit of using Reducer Hook is that you can apply custom logic within the reducer before updating the state. React garanties the dispatch function is not going to change after a component’s re-render.
The syntax of this React Hook is familiar to the one used with Redux. Here is a list of concepts that need to be understood before using the useReducer
Hook in React:
useReducer
that you should call to apply changes to the state.The initial value of the state can be passed in the useReducer
hook as the second parameter. In case you want a lazy loading of the state, the third parameter accepts a callback function triggered when the hook initialises.
Here is an example of code using the React Effect Hook.
function reducer (state, action) {
return action.type === "sum" ? state+1 : state;
}
const [state, dispatch] = useReducer(reducer, 0);
<button onClick={()=>{dispatch({"type":"sum"})}}>Sum one</button>
<button onClick={()=>{dispatch({"type":null})}}>Stay as is</button>
ReactJS allows you to set up application contexts. React Context Hook is used to access the properties of the current context. This allows to set up state configurations without passing them around the component tree. Here are the steps to create a React Context:
const context = React.createContext(config)
, where config
is the object created on step 1.
. Here you need to specify which configuration of your context is going to be used.useContext
hook.Here is an example of code using the React Context Hook.
// Component A
const theme = {
light: {
title: "I am in light theme"
},
dark: {
title: "I am in dark theme"
}
}
export const ThemeContext = React.createContext(theme);
<ThemeContext.Provider value={theme.dark}>
<App />
</ThemeContext.Provider>
// Component B
const theme = useContext(ThemeContext);
<h1>{theme.title}</h1>
What are the top JavaScript Frameworks that you should be looking for in 2021? This article provides a list of the most commont frontend frameworks to use on a JavaScrtipt project.
Updating Angular projects on a regular basis is very important. This article covers how to update Angular to version 11 from version 7. It also provides information about new features on Angular 11.
A basic overview of Redux, an open-source JavaScript library for managing and updating an application state. A dive into its actions, APIs and tools for state management of modern applications.